4 minutes
How I made 10 line ruby script to get my 1st jab done! 🤷🏼
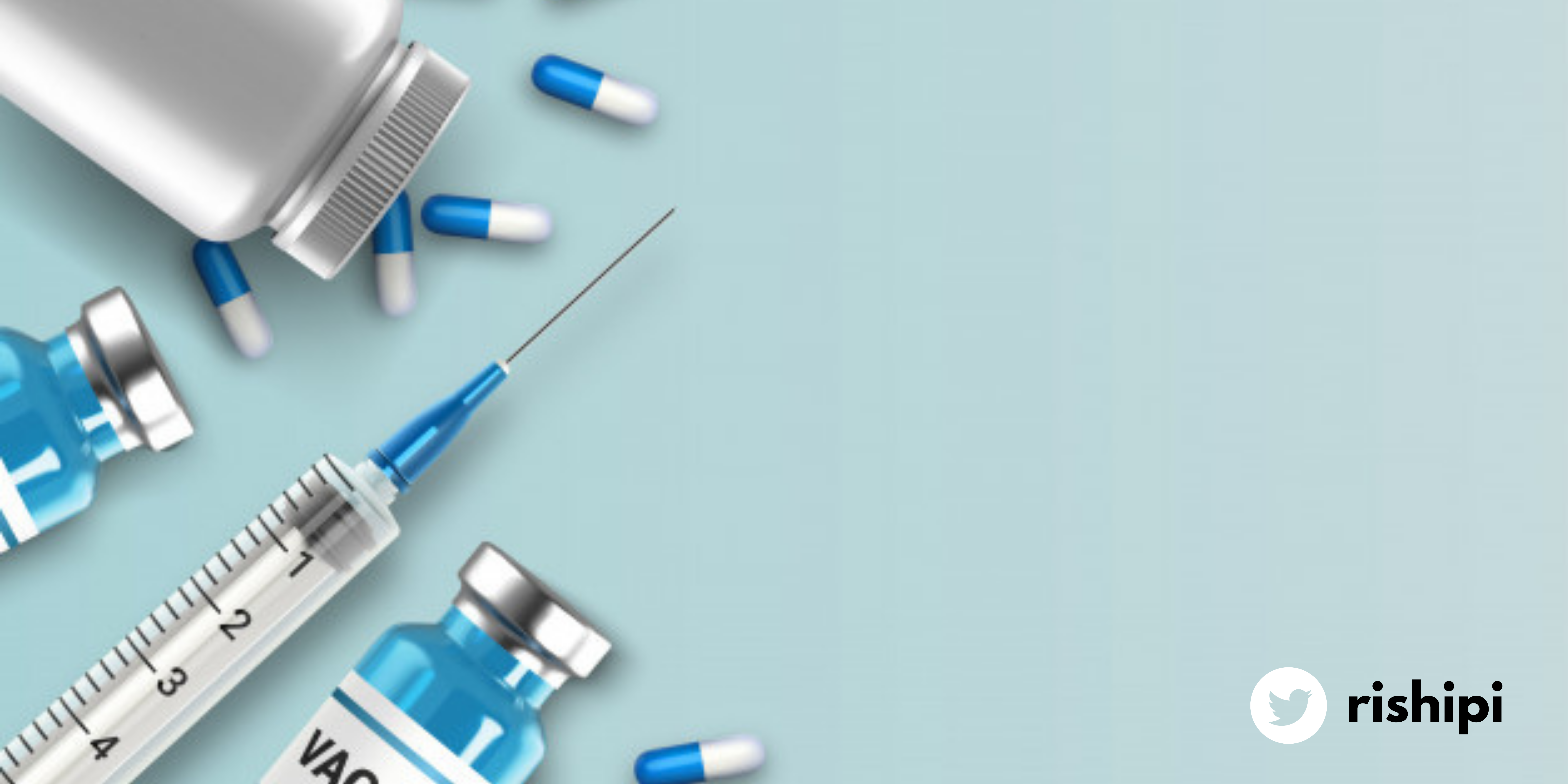
Recently, the Indian govt announced vaccination for 18-45 age group from 1st May ✨
Anyone who wants to vaccinate in this age group can visit official website or install Arogya Setu App from Google Play to book a slot for vaccination.
The problem is vaccination is limited and slots are also limited, so whenever anyone wanted to book a slot, it always shows booked on official website 😢
After visiting website multiple times in a day to book my slot, I was unable to find any available slots because it always shows booked 🥺
Now, on the same website when you click on a search to find slots, it calls one API which is used to get availability of slots for selected district -
Cowin already have Public APIs available, which anyone can use to check slots availability.
Out of all public APIs, I used calendarByDistrict
API which returns planned vaccination sessions for 7 days from a specific date for a given district.
Which returns response that contains all required information like center details, session details and available slot details 🙌🏼
{
"centers": [
{
"center_id": 1234,
"name": "District General Hostpital",
"state_name": "Maharashtra",
"district_name": "Pune",
"pincode": "411057",
"lat": 28.7,
"long": 77.1,
"from": "09:00:00",
"to": "18:00:00",
"fee_type": "Free",
"sessions": [
{
"session_id": "3fa85f64-5717-4562-b3fc-2c963f66afa6",
"date": "10-05-2021",
"available_capacity": 50,
"min_age_limit": 18,
"vaccine": "COVISHIELD",
"slots": [
"FORENOON",
"AFTERNOON"
]
}
]
}
]
}
You may visit this link to get an idea about detailed response here.
For sending web requests in ruby language, Net::HTTP
class available. For above request, you can execute below code in your rails console for getting center list JSON -
# District - Pune, Date 10th May, 2021. You can modify it as per your requirement
uri = URI.parse("https://cdn-api.co-vin.in/api/v2/appointment/sessions/public/calendarByDistrict?district_id=363&date=10-05-2021")
results = Net::HTTP.get(uri)
results = JSON.parse(results).with_indifferent_access
It'll give you a list of centers like this in your rails console.
Now we have results
hash variable available in which root element is centers
like this -
{
"centers": [
{
"center_id": 1234,
"name": "District General Hostpital"
"sessions": [
{
"date": "10-05-2021",
"available_capacity": 50,
"min_age_limit": 18
}
]
}
]
}
Basically, from above data, we need to compare following attributes to get available slots -
min_age_limit
attribute comparison with18
(min_age_limit == 18
)available_capacity
is greater than0
(available_capacity > 0
)
Alright, let's search for available slots then -
availability = {}
results[:centers].each do |center|
# 1st condition - Checking Age
sessions = center[:sessions].select{ |c| c[:min_age_limit] == 18 }
# 2nd condition - Checking Availability
sessions_with_availability = sessions.select{ |s| s[:available_capacity] > 0 }
# Collect date for specific pincode if there's vaccine availability
availability[center[:pincode]] = sessions_with_availability.collect{ |s| s[:date] } if sessions_with_availability.any?
end
That's it 🥳 availability
variable will have following hash value if any availability found.
❯ availability
❯ {413102=>["02-05-2021"], 411044=>["02-05-2021"]}
It contains dates on which slots available for particular Pincode 🔖
Now, as we have all required data with us, we can do following thing with this script -
- Write a fancy output, maybe create mail/SMS to send notification.
- Create a cron-job to run it every 1 minute and notify if there's avaibility.
Apart from this, you may also create a minimal rails app to get user details from users and send notifications to help them find available slots -
Conclusion
Automation is good sometimes if used properly. In this case also, if you wanted to run your cron-job in large intervals, say once in 10 minutes, it's fine.
But, if you run it on a very small interval and access cowin API frequently, there might be a chance that your IP might be blocked to access those API and you're no more able to access it. So use it wisely ✌🏼
Important
Sometime you'll get following error while API call -
We can't connect to the server for this app or website at this time. There might be too much traffic or a configuration error. Try again later, or contact the app or website owner.
It might possible that there is lots of traffic on server on pick hours, execute API after some time(approx 1 hour), so that it'll give list of centers.
Reference
If you found this article insightful and helpful, then do let me know your views in the comments 🙂
In case you want to connect with me, here's my twitter - rishipi