One minute
Validate keys of hash using assert_valid_keys 🚧
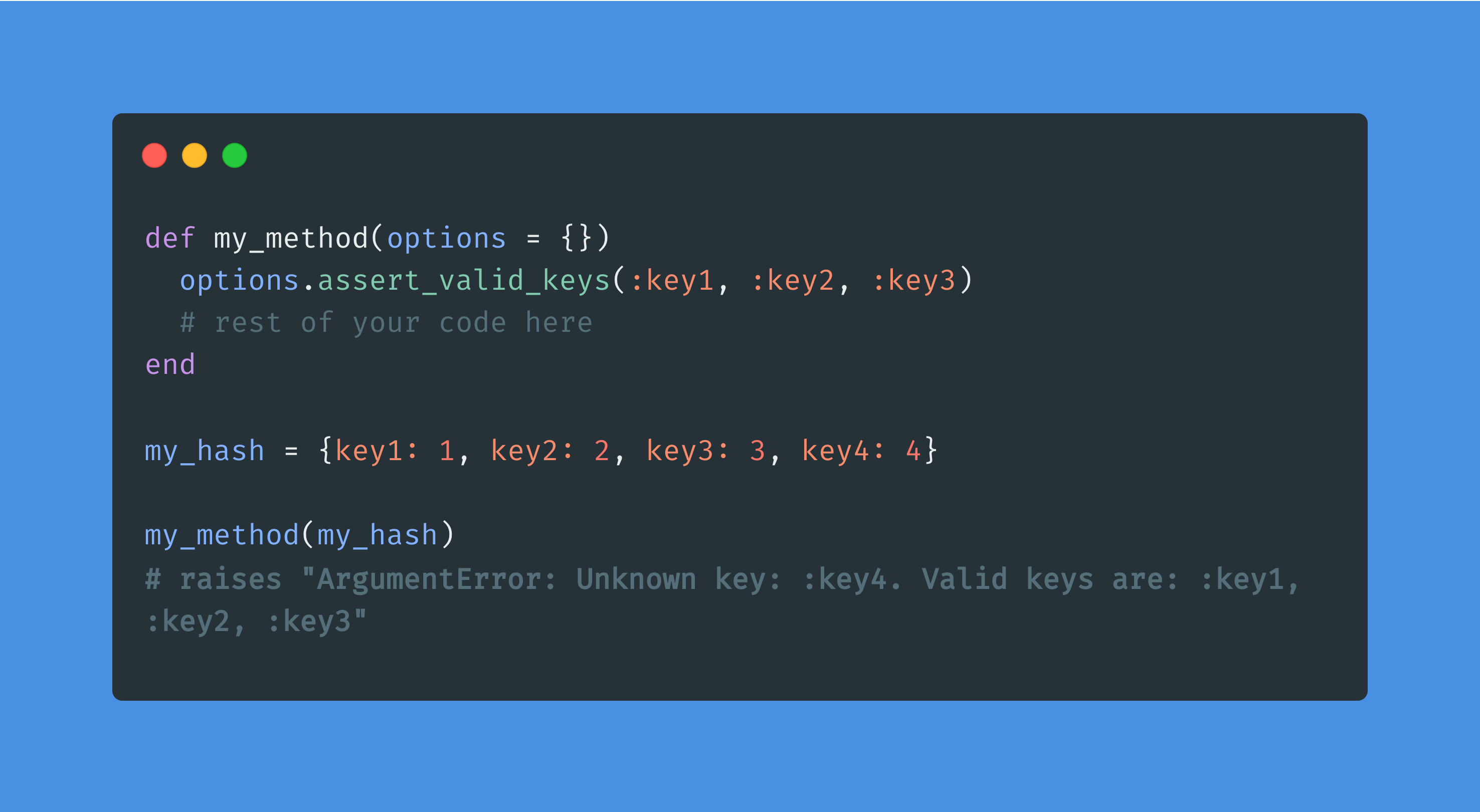
Have you heard of the assert_valid_keys
method for the Hash class in Rails?
This method provides an easy way to validate the keys of a hash against a list of expected keys. It raises an error if the hash contains any keys that are not included in the expected list.
This is useful for ensuring that only valid parameters are passed to methods and avoiding unintended behavior.
Here's a code snippet showing how to use assert_valid_keys
:
def my_method(options = {})
options.assert_valid_keys(:key1, :key2, :key3)
# rest of your code here
end
my_hash = {key1: 1, key2: 2, key3: 3, key4: 4}
my_method(my_hash)
# raises "ArgumentError: Unknown key: :key4. Valid keys are: :key1, :key2, :key3"
This method can help you write more secure and maintainable code by catching mistakes early in the development process. Give it a try and let me know what you think!
Read other posts