2 minutes
Tweaking into whenever gem timezone ⏳
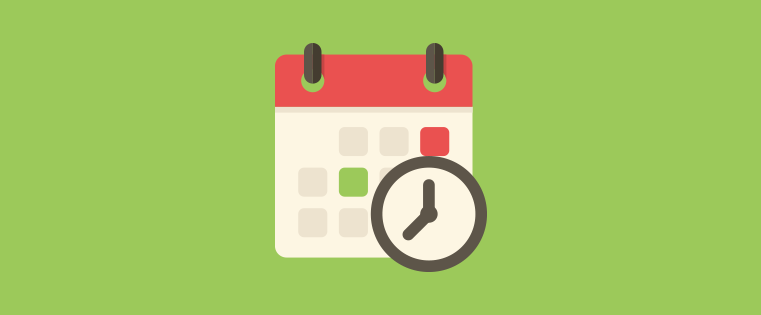
whenever gem is used when there’s need of run specific job at particular intervals, say -
- send invoices
- send daily reports
- send reminder notifications etc..
Once you setup whenever, it will create a schedule.rb file under config directory. You can edit it to schedule particular task. Suppose we need to send daily sales reports at 9:00 AM every day:
# Send daily sales report at 9:00 am everyday.
every 1.day, at: '9:00 am' do
rake 'send_daily_sales_report'
end
Unfortunately, it becomes more complicated as every-time we need to convert hours to UTC to add it in our schedule.rb file 😖
Now, to solve this we can do one thing, we can convert local time to UTC using following method -
❯ ActiveSupport::TimeZone.new(‘Mumbai’).local_to_utc(Time.parse(‘9:00 am’)).strftime(‘%l:%M %P’).strip
"3:30 am"
It’ll convert local time to UTC time(used by server). We can create a separate method in schedule.rb file which include above statement.
require File.expand_path(‘../config/environment’, __dir__)
# Convert IST to UTC and return array of UTC time like ['2:30 am', '3:30 am',...]
def to_utc(*time)
begin
time.collect{ |t| ActiveSupport::TimeZone.new('Mumbai').local_to_utc(Time.parse(t)).strftime('%l:%M %P').strip }
rescue Exception => e
Rails.logger.info "Error: #{e.message}"
Rails.logger.info "Backtrace: #{e.backtrace}"
end
end
# to run the cron in 10 to 7 IST timings.
every 1.hour, at: to_utc('10:00 am', '11:00 am', '12:00 pm', '1:00 pm', '2:00 pm', '3:00 pm', '4:00 pm', '5:00 pm', '6:00 pm', '7:00 pm') do
runner 'UtilitiesService.clean_logs()'
end
Now, your code become more readable as to_utc
method is available for converting IST time to UTC server time 🤟🏼
Setting application timezone should be part of whenever gem. It’s not available as of now and there’s open issue also available on it’s github repository -
Can whenever take timezone from config? #662 🛠
I hope it might available in near future 🤞🏼
References -